Overview
If you’re just tuning in, welcome to the Learn C Programming Series! We covered how to setup a programming environment in the last post, so be sure to check that out if you don’t have one setup. Otherwise, let’s get coding.
If you find this post helpful, be sure to share it with a friend that would find this useful.
What is a Hello World?
A Hello World/Hello World project is a simple program that prints something out to the terminal. This way, you can put on your resume that you are “proficient” in the programming language. But by the end of this course, you’ll be comfortable programming in C
In your text editor, type out the following code:
#include <stdio.h>
int main(void)
{
printf("Hello World!\n");
return 0;
}
We need to get our print function from the stdio.h library, so we include it at the top of our code. Additionally, we need to have some entry point into our code. In C, this is our main function. Within our main function, we can use printf to print out information onto the terminal screen.
You may notice that we include a \n immediately after World! . This is a newline character. It allows us to specify to the system that are done printing on this line and to print out the next set of information on the following line below -just like a typewriter. While optional, print formatting makes it easier to read what we display to the screen.
Additionally, it is best practice to include return 0 at the end of our main function. Programs have a return code to indicate if they finished properly, with 0 indicating that it ran with zero errors. Since the main function will house all of our logic, it is proper to end our program by returning 0 back to the system.
Run this in your environment by using the following commands in your terminal:
gcc [name_of_program].c
./a.out
GCC stands for General C Compiler. It takes your code and translates it into a format that your system can read. This output is stored in a file called a.out. The second command will access this file and run the program on your computer.
Once you are completed with this exercise, complete the assessment before moving on to the next lesson.
That's Cool Jacob, But How Do I Make Something Cool with C?
Trust me, it gets cooler! Now that we can print stuff to the screen in the C language, let’s work on using integers and floats. The C programming language has a very simple set of rules for writing equations and storing results from basic math equations:
Variables allow us to specify values in our program that we can reference throughout our code. We can think of variables as buckets or placeholders for our code.
- Buckets have a limited space -each variable we declare in C will have a fixed size.
- Buckets have a unique shape -variables are designated to store integers, decimals, characters or pointers.
- We do not have an infinite supply of buckets -the more variables we introduce into our program, the more RAM it will take to function. This is not much of a concern with advances in computing, but it is worth noting.
Declaring Variables and Instantiation
Using the same analogy, we can allocate space in our program to fit buckets that we will need in order to do our computation. This step is called declaration. We can think of this step like we are taking publicly used buckets on the beach for our sand castle project. This way, no one else comes and takes our buckets until we are done using them. When our program closes, we will give back this buckets back so that others may use them. Tying it all together, we can request more space from the system for variables and give this space back when we are finished with the program.
There are two components to declaring a variable: the variable type and name. The variable type defines what kind of variable we would like. There are a variety of types we can declare -each with a fixed allotted space. The variable name is what we will use to reference this space. In doing so, C can support multiple variables of the same type, however, each must have a unique name like so:
int a;
double b;
double c;
float f;
char d;
char *e;
We have multiple variables declared. a is an integer which can store integers. b and c are both double variables. Doubles and floats can be used to store floating point numbers (or numbers with decimal points). Doubles get their name because they are double the size of floats. Variable d is a character variable that stores one character. e is a fixed string, meaning it can store one string (multiple characters put together), however the only way to change this value is to provide a new string.
The C language supports a couple of funky variable naming conventions, but in general we will use the following guidelines in this course for naming variables:
- Variables should start with a lowercase letter (the * in some variables are included in the type and do not count towards the name.)
- Variables describe what their purpose our in a short and sweet manner
- Variables are snaked_cased_like_this or camelCasedLikeThis (pick one and stick with it in your program)
- Variables can have numbers after the first letter like so: i1, i2, i3.
Instantiation
We have our buckets we can work with, however, they do not have much meaning to us at the moment. Since our buckets are publicly used, there is no telling what the previous user did with them and if they left anything there. Similarly, the space we get from our system will have random values. It is important to instantiate your variables, or give them a starting value. We can do this in the following manner:
int my_favorite_number;
my_favorite_number = 0;
We can change the value of our variable later in the code like so:
my_favorite_number = 4;
Since we’ve already declared that my_favorite_number is an integer variable, we do not need to put int in front of it afterwards; the program just needs the name of our variables after declaration.
If we know the value we want our variable to be at the time of declaration, we can do a declaration/instantiation like so:
int my_favorite_number = 4;
This applies to all variable types! Copy the following code into your terminal and give it a try:
#include <stdio.h>
int main(void)
{
int a = 1;
double pi = 3.14;
char *string = "Hello World!";
char c = 'C';
// Printing our variables
printf("a = %d\n", a);
printf("pi = %f\n", pi);
printf("%s\n", string);
printf("My char is %c\n", c);
return 0;
}
You can always change the value stored in your variable after you instantiate it. This is called an assignment or reassignment operation.
Print Formatting
You may have noticed a couple of weird characters in the print statements. Those are format specifiers. This tells our compiler that we are going to print out the values stored in our variable. To do this in C, we specify what type of information we will be printing. After our print statement, we include our variable that we would like to print. The type specified should match the variable you enter at the end of the string.
- %d: integers
- %f: floating point numbers
- %c: characters
- %s: strings
- %p: pointers (more on this later)
You can have multiple variables printed in the same print statement. They can also have different data types too -just be sure you don’t mix them up.
#include <stdio.h>
int main(void)
{
int a = 1;
int b = 2;
double c = 3.0;
printf("%d %d %f\n", a, b, c);
}
You Said 3 Programs, Where's The Third C Program?
Ask and you shall receive, but you don’t have to be so mean about it… Let’s reinforce some more arithmetic operations before we get too far ahead of ourselves. There are several operations, shorthands and “math rules” to consider when programming. We’ll dive into the details for implementing math into your C programs.
Integer Arithmetic
There are a variety of operators you can use to manipulate numerical variables (integers and floats). We can do addition, subtraction, multiplication, division and modulo (remainder of division) operations. These are particularly useful in creating smarter programs.
int a = 10;
int b = 20;
int c;
c = a + b;
printf("c = %d\n", c);
The above sample is an example of addition. We can take two numbers add them and store the result in another variable. We use the variable c to find out the value of this arithmetic in the following print statement. If we wanted to simplify this even further, we can do the following:
int a = 10;
int b = 20;
printf("Sum = %d\n", a * b);
So long as the final value is still an integer value, we can have expressions as our value in the printf() ranging from longer complex computation or even function calls which we will get into later on in the course. Pass some value to the sample code in your workspace to see how arithmetic works in more detail:
#include <stdio.h>
int main(void)
{
int a = 1;
int b = 0;
printf("Arithmetic Computation\n");
printf("a + b = %d\n", a + b);
printf("a - b = %d\n", a - b);
printf("a * b = %d\n", a * b);
printf("a / b = %d\n", a / b);
printf("a % b = %d\n", a % b);
return 0;
}
You may have noticed an error. This is a divide by zero error. Much like in traditional mathematics, dividing or modding by zero gives an undefined result. If you do this in C, it will either prevent you from compiling the code or let you run it and crash. See if you can find a way to make the code above work.
Floating Point Arithmetic
The above arithmetic will actually work on all floating point numbers!
include <stdio.h>
int main(void)
{
double a = 1.0;
double b = 0.0;
printf("Arithmetic Computation\n");
printf("a + b = %f\n", a + b);
printf("a - b = %f\n", a - b);
printf("a * b = %f\n", a * b);
printf("a / b = %f\n", a / b);
printf("a % b = %f\n", a % b);
return 0;
}
You’ll notice that some precision may be lost when using floating point numbers. This is because of the schema used to store decimal numbers. If we stored every digit in the decimal, it would significantly increase the amount of memory needed to support decimal numbers. The floating point numbers take an approximation of what is entered to save on memory. We will need to keep this in mind when we discuss making comparisons between variables.
Shorthands
Consider the following code:
int a = 10;
a = a + 10;
a = a + 10;
printf("a = %d\n", a);
In the code, we declare and instantiate a. We then add 10 to a and store the result back into a. We repeat this and print out the result, which should be 30.
In instances like these, we can actually shorten these expressions:
int a + 10;
a += 20;
a -= 10;
a *= 2;
a /= 3;
a %= 5;
printf("a = %d\n", a);
The same also applies to all arithmetic expressions and floating point numbers! We can also use prefix and postfix addition and subtraction on variables to add or subtract 1 from the stored value like so:
int a = 0;
++a;
a--;
printf("a = %d\n", a);
Prefix means it will add one at the beginning of the operation. Postfix means it will add one after the operation. This is particularly useful for ensuring the correct order of operations. To specify order of operations, we can use parentheses or rely on the language’s own order of operations. In C, order of operations are as follows:
1.) Parentheses
2.) Multiplication, Division, Modulo
3.) Addition, Subtraction
4.) Assignments and Compound Assignments (Shorthands)
Let's See This In Action
Now that we know a little more about the C programming language, let’s dive into our first example in the series:
Wrapping It All Up
Now that we know how to use numbers in our C program, we can introduce logic. Logic is one of the core functions in any program, otherwise our programs would be pretty boring.
Thank you for reading all the way down here! I hope this information was useful and stay tuned for the next installation where we cover conditional logic in the C programming language.
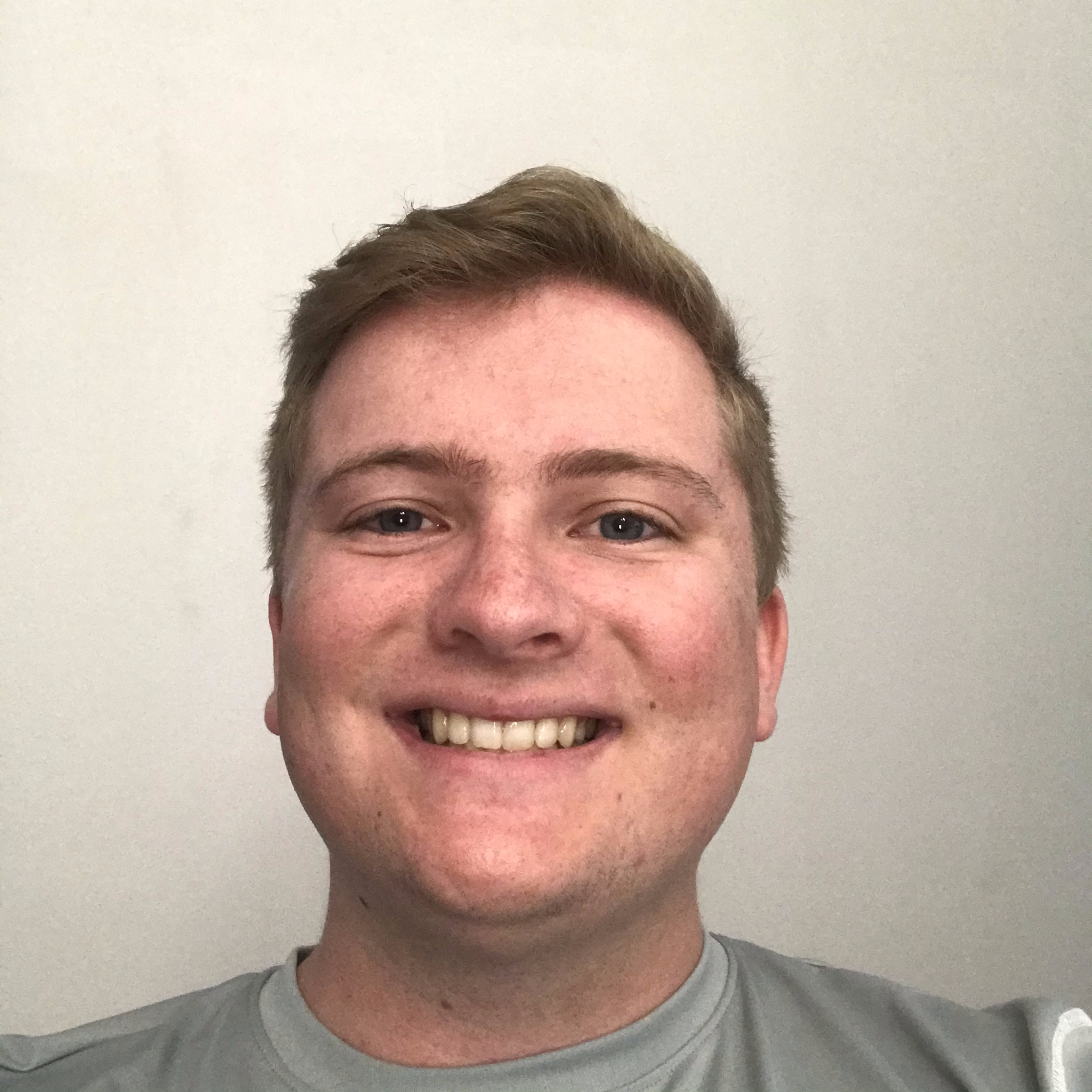
Founder and CEO of R U Coding Me LLC. Jacob obtained his Bachelor’s of Computer Science at the University of Central Florida. He likes to go to the gym and teach people about technology.