What is Debugging?
Overview of Debugging
Debugging is an art form, rather than a science. Debugging is the act of removing any issues that you may have in your code. The term was coined back in the day when they wrote programs on paper sheets! Some programmers at Harvard found a moth in their system that processed the paper sheet programs and had to debug the system in order. Let’s go over some of the common errors in programming:
Syntax Errors
Syntax errors occur when a program is not in specification with the programming language. In other words, there is an illegal expression or typo that is preventing the compiler from understanding your program. These will always prevent your program from running and are typically easy to diagnose.
Semantic Errors
Semantic errors occur when your program does one thing when it should be doing another. For instance, if your mathematic logic does not result in the expected expressions or if print out information to the screen and it does not print correctly. These errors can be tricky, but with enough patience and technique can be solved.
Stages of Debugging
There are a variety of different philosophies on debugging. Most professional development environments will use expensive debugging software. We will use open-source tools built in with C to diagnose all of our problems instead! But before we bring out the big guns, we can sometimes solve problems ourselves without using a debugger.
- Isolate the problem: As we test our code, we can check for segmentation faults, dumped cores or incorrect output. We have to identify the smallest portion of our code that we think is the problem before proceeding; as this will make life easier.
- Test multiple solutions: With the code isolated, test out a couple of different things in an attempt to break it. Understand why it is broken and how to code up defenses against the cases that break your code.
- Reintegrate with the program: Once you have solved the problem, reintegrate your code with the larger program. Sometimes there are issues with integrating components together and they are only evident once you confirm that the individual components are working fine.
- Keep working your way up the code: You will eventually find the problem and solve it, just give it some time! When your programs exceed a few hundred lines of code, it may be beneficial to use the GDB or general debugger.
As we get into more sophisticated content later this series, we’ll need to use these tools to efficiently solve our issues. GDB (general debugger) is the default software that ships with C. This command line tool will iterate through the program execution and tell you where you crash (if you crash). Otherwise, the program will terminate successfully. Remember how we use return 0; at the end of our main() function?
While we have you here, we might as well talk about Valgrind. Valgrind is an awesome tool to detect memory leaks associated with Dynamic Memory Allocation. If you are unfamiliar with this concept, do not worry! We’ll be covering DMA and how to properly employ it in the C language, so be sure to stick around for this post.
Memory leaks are caused when you allocate areas of RAM and do not give them back to the system. Little leaks here and there do not particularly matter, but overtime can stack up. If you have ever played an intense video game over a long period of time, you may have noticed a performance drop as you continued to play the game. Odds are, the game might have a memory leak or two that slowly ate away at your RAM.
To get your RAM back, just restart your computer.
How To Employ These Tools
When your program doesn’t work, consult the check list. Once you can isolate your problem, you can begin implementing a solution. With GDB, you can find out where your code crashes and work your way to this point. Stepping through GDB is another handy way of measuring how your program is performing and where it crashes. It takes time to get used to this, but it is an invaluable skill to have. Once you know how to use a command line debugger, the graphical debuggers will feel like a treat!
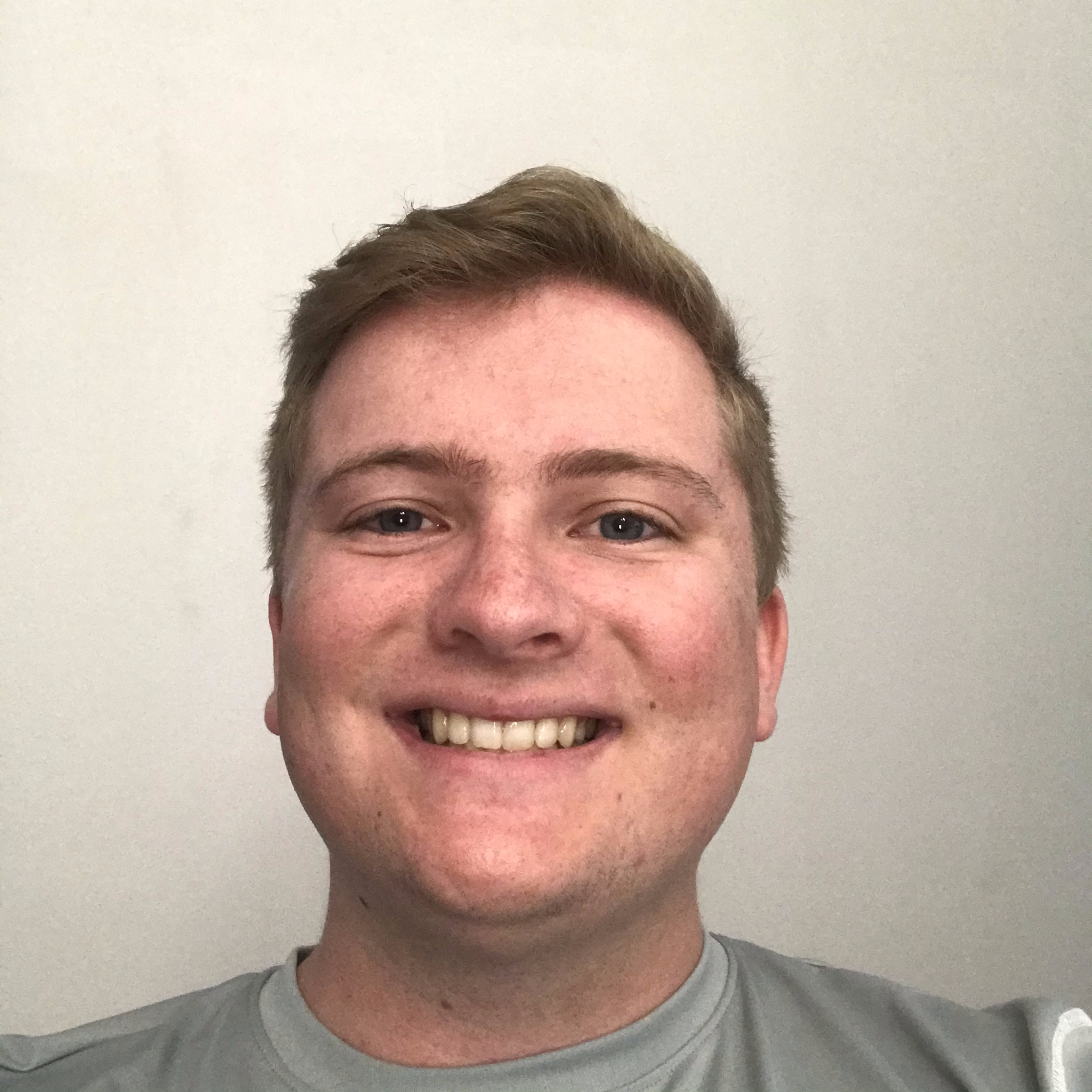
Founder and CEO of R U Coding Me LLC. Jacob obtained his Bachelor’s of Computer Science at the University of Central Florida. He likes to go to the gym and teach people about technology.